Controlling form tabbing with jQuery
Update: Paceville.com is now online, where the effect described in this tutorial can be seen.
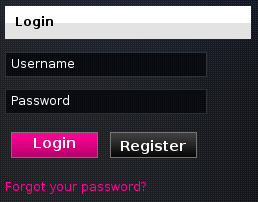
Take a look at the image to the right, a classic example, due to space restrictions we have a login form – that has the information we would normally put in labels – as values instead.
The first requirement is that as soon as a field gets focus its contents should be nulled, the second is that the password field should of course be a password field when getting focus, and the third is that everything should be tabbable.
So I implemented #1 and #2, but for some reason the form would not tab properly in for instance Chrome and Safari, it worked just fine in Firefox 3.x.
Let’s take a look at the complete solution:
jQuery(document).ready(function(){
jQuery("#mod_search_searchword").focus(function(){
jQuery(this).val('');
});
jQuery("#mod_login_username").focus(function(){
jQuery(this).val('');
jQuery(this).keydown(function(event){
if(event.keyCode == 9 || event.keyCode == 11)
jQuery("#mod_login_password").trigger('focus');
});
});
jQuery("#mod_login_password").focus(function(){
jQuery(this).replaceWith('<input id="mod_login_password" class="text_input" type="password" value="" name="passwd"/>');
});
});
Note the use of jQuery instead of $, we’re working with Joomla here which is using Mootools so we don’t want any conflicts, that’s why.
Let’s discuss the password field first (#mod_login_password). On focus we replace it with the argument string. I couldn’t figure out how to change just the attribute here, doing attr(“type”, “password”) didn’t work for me, that’s why I ended up with this less than ideal solution.
Anyway when the username field gets the focus we zero out its contents and then listen for all key presses, if we have a vertical or horizontal tab (I’ve got no idea if both cases are needed but just in case since I don’t know the difference or the history behind there being two types) we trigger a focus event on the password field.
Related Posts
Tags: Javascript, Joomla, jquery